Scripting Acorn¶
See also: Example AppleScript and JavaScript Scripts
You can script in Acorn using a couple of different languages- AppleScript, or Cocoa Script (which is pretty much JavaScript with some extra goodies). You can edit Cocoa Script scripts using TextEdit- but you might have an easier time using something like Apple's Xcode (which is free), BBEdit, SubEthaEdit, or even TextMate. These text editors offer niceties such as syntax coloring and line numbers, making it easier to write and debug your scripts.
If you don't know JavaScript, there are lots and lots of tutorials out on the web you should check out.
Scripting Acorn with AppleScript can be done from the AppleScript Editor, located in your Applications folder.
Writing plugins for Acorn in JavaScript¶
Here is an example for a quickly resizing your image in Acorn
Paste that into a next text file in your favorite editor, and save it to file named "Resize to 50%.js" in Acorn's Plug-Ins folder (which you can get to by choosing the Help ▸ Open Acorn's App Support Folder). Restart Acorn and choose the menu item Filter ▸ Resize to 50%. Your image should then resize to 50% of its original size.
So what is going on here?
Well, first off- whenever you save a file in your Acorn Plug-Ins folder, and it has the file extension "js" - it will show up in the Filter menu. Then, when the plugin is called, Acorn looks specifically for the function named "main" in your image, and then passes it a copy of the current layer's image. Nothing was done directly with the image. Instead Acorn was asked for the current document, and then resized it like in the previous script.
Here is another example. This can go in a new file named "Resize Layer to 50%.js", in the same Plug-Ins folder as before (and yes, you'll need to quit and restart Acorn for the plugin to show up):
In this example, something is actually done with our new image (which is a CIImage by the way). A new image is made by applying a transform to the original image, which scales it by 50%. Return to that image. You'll notice that when calling this function your layer has been replaced with a new layer, which is 50% of the original size. However, your overall image size has not changed because you didn't change the document's size.
Before this plugin:
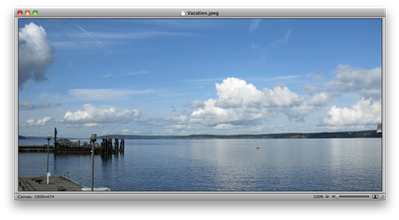
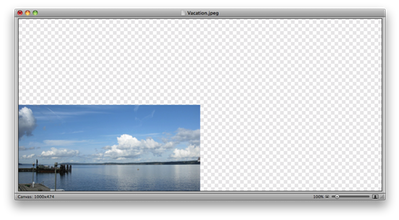
Scripting Acorn with JavaScript and Automator¶
Acorn 6 and later comes with an Automator action to run JavaScript in Acorn. Here's an example that will open up passed files, and saving them as PNGs.
The possibilities are endless.
If you would like to see a specific example, or you have a task that you would like to see some example code for contact: support@flyingmeat.com and we'll see if we can come up with a solution for you!
Scripting Acorn with AppleScript and Automator¶
Here is an example Automator action that will take the images passed to it, and web export them to PNG files.
Note For App Store Users.
If you've purchased Acorn from the Mac App Store, certain AppleScripts, Automator Actions, and JavaScript scripts may not work for you because of sandboxing restrictions required by Apple. The direct version of Acorn does not have these issues.